How to get language and region names for various translations in JS?
โ2 min read
- Internationalization
- Intl
- Translating websites
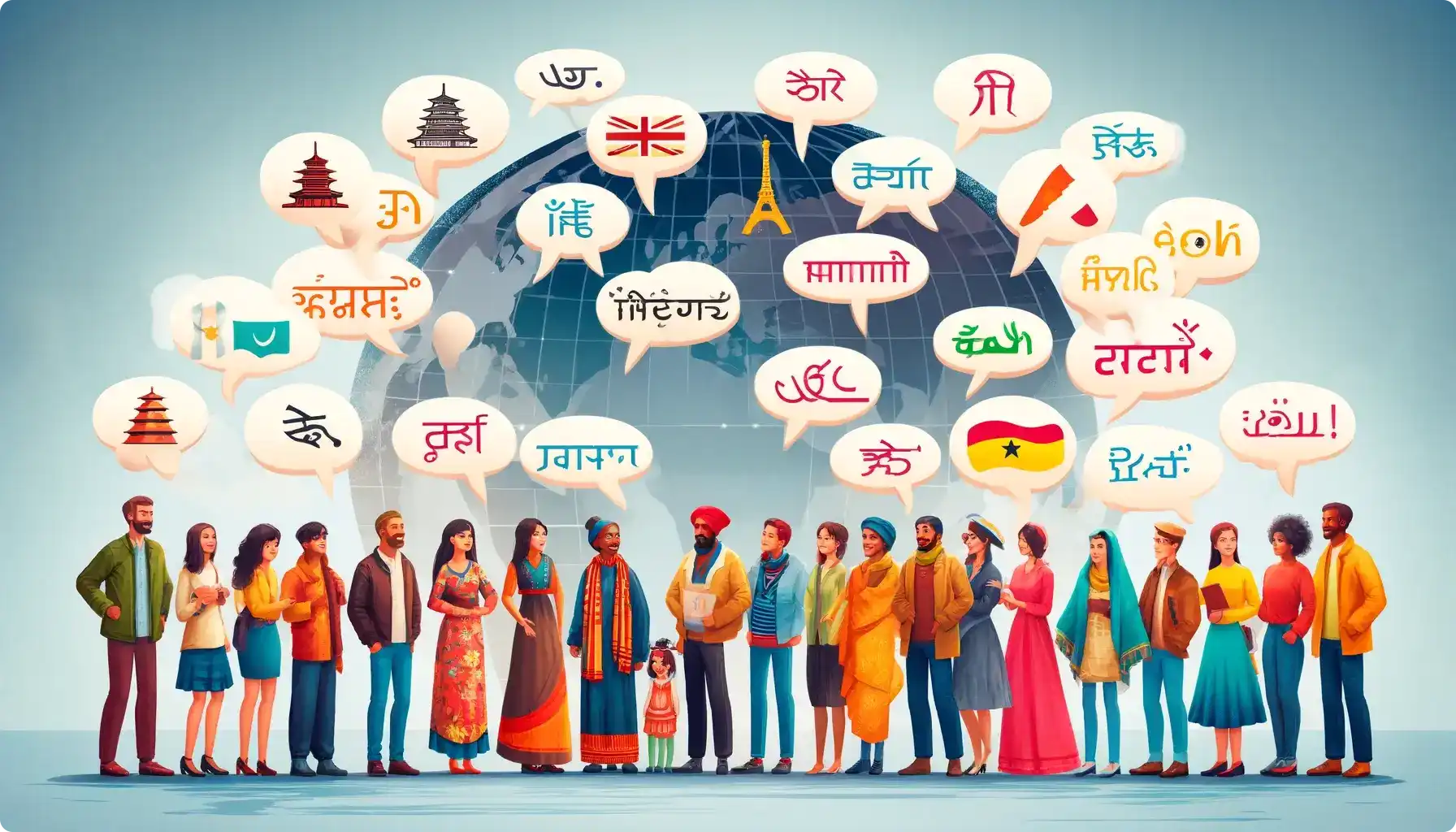
Let's say you want to your current user's region name in their native language
For English it would be 'United States', for French 'รtats-Unis', for German 'Vereinigte Staaten' and so on.
So what to do now?
Create an object with each translation there is? Poor idea.
Ask GPT-4 API to translate items? Better, but also far from ideal.
Use the Intl
Intl nowadays consists of curated map of translations for each locale. For following items language
, region
, script
, currency
, calendar
, and dateTimeField
you can get a well made list of translations without using third party at all.
Get regions in user's browser language
// these probably would come from backend side of things to be configurable
const localesToTranslate = ["US", "FR", "ES", "DE"] as const;
// any other locale can be passed as baseline for translations
const regionNames = new Intl.DisplayNames(navigator.language, {type: "region"});
const regionsInBrowsersLanguage = localesToTranslate.map(ltt => regionNames.of(ltt));
console.log(regionsInBrowsersLanguage);
// result in Polish-> ['Stany Zjednoczone', 'Francja', 'Hiszpania', 'Niemcy']
Get regions in Spanish
const regionNamesES = new Intl.DisplayNames("ES", {type: "region"});
const regionsInSpanish = localesToTranslate.map(ltt => regionNamesES.of(ltt));
console.log(regionsInSpanish)
// result in Spanish -> ย ['Estados Unidos', 'Francia', 'Espaรฑa', 'Alemania']
Get currencies in Spanish
// let's use type currency this time, since three items share the same currency
// there are only two left ๐๐๐
const currencyNamesES = new Intl.DisplayNames("ES", {type: "currency"});
const currenciesToTranslate = ["USD", "EUR"] as const;
const currenciesInSpanish = currenciesToTranslate.map(ctt => currencyNamesES.of(ctt));
console.log(currenciesInSpanish);
// result in Spanish -> ['dรณlar estadounidense', 'euro']
Translate smart
Not everybody is familiar with the Intl library and it is indeed a very powerful tool to make our app accessible for anyone in the world. Have a great day, see you on the next one ๐.